Have you even worked on a team of software developers and the code is absolutely horrible. We are talking spaghetti code and inefficient code like nested loops, which is a big no-no according to Big-O.
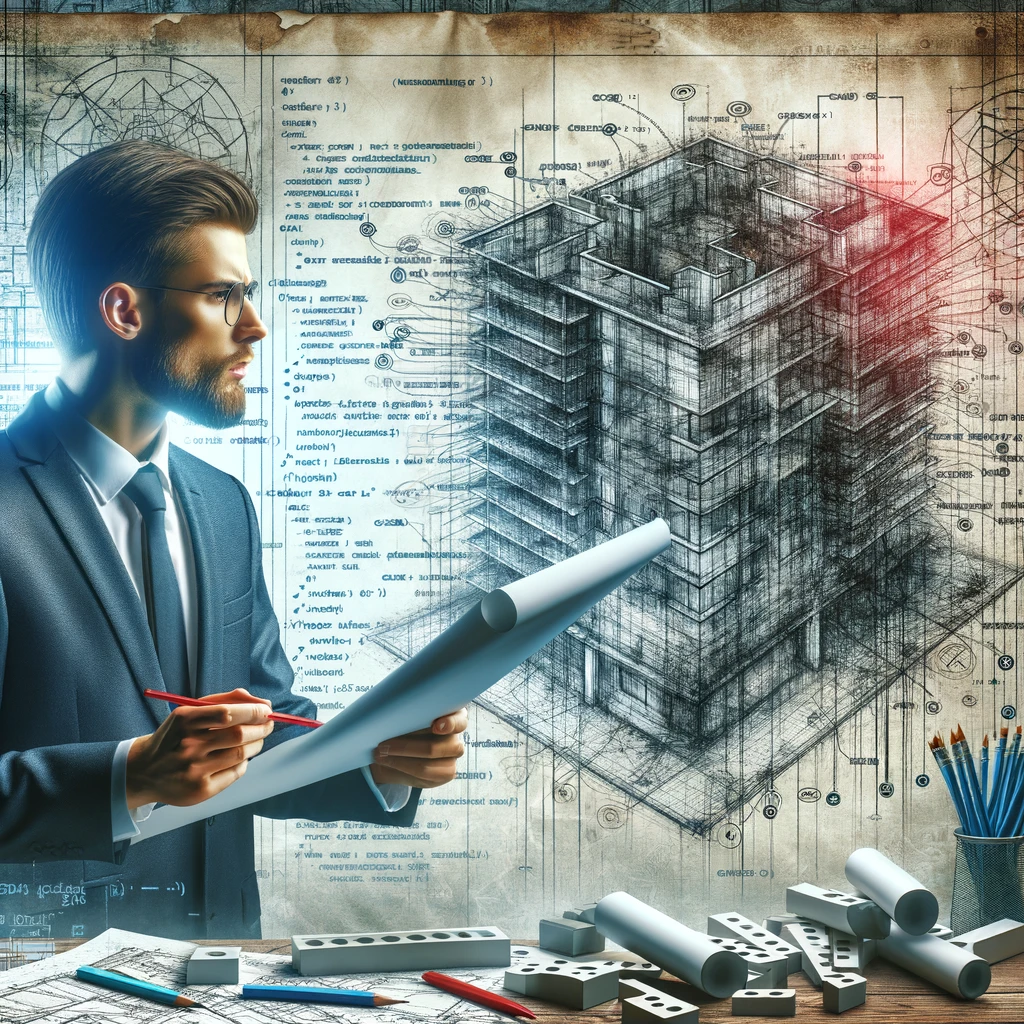
As we write software applications, it is important to tackle the problem of writing messy code so that it is easy to understand. I’m talking about code that is so bad that it gives you a headache trying to figure it out.
Why bother with refactoring? Software, much like a building, needs maintenance to remain functional and efficient. Over time, additions and modifications can lead to a codebase that’s difficult to read and expensive to change. Refactoring addresses these issues by improving the nonfunctional attributes of the software. This, in turn, leads to enhanced code quality and easier maintenance down the line.
In the following sections, I’ll share with you an example of spaghetti code and a toolkit for code refactoring best practices. These techniques will not only make your code more elegant, but they’ll also foster an environment where future changes can be implemented with greater ease and less risk of introducing bugs.
Spaghetti Code Example
I’m a fan of Italian food, but when it comes to coding, Italian food and spaghetti just don’t blend well together! 😄
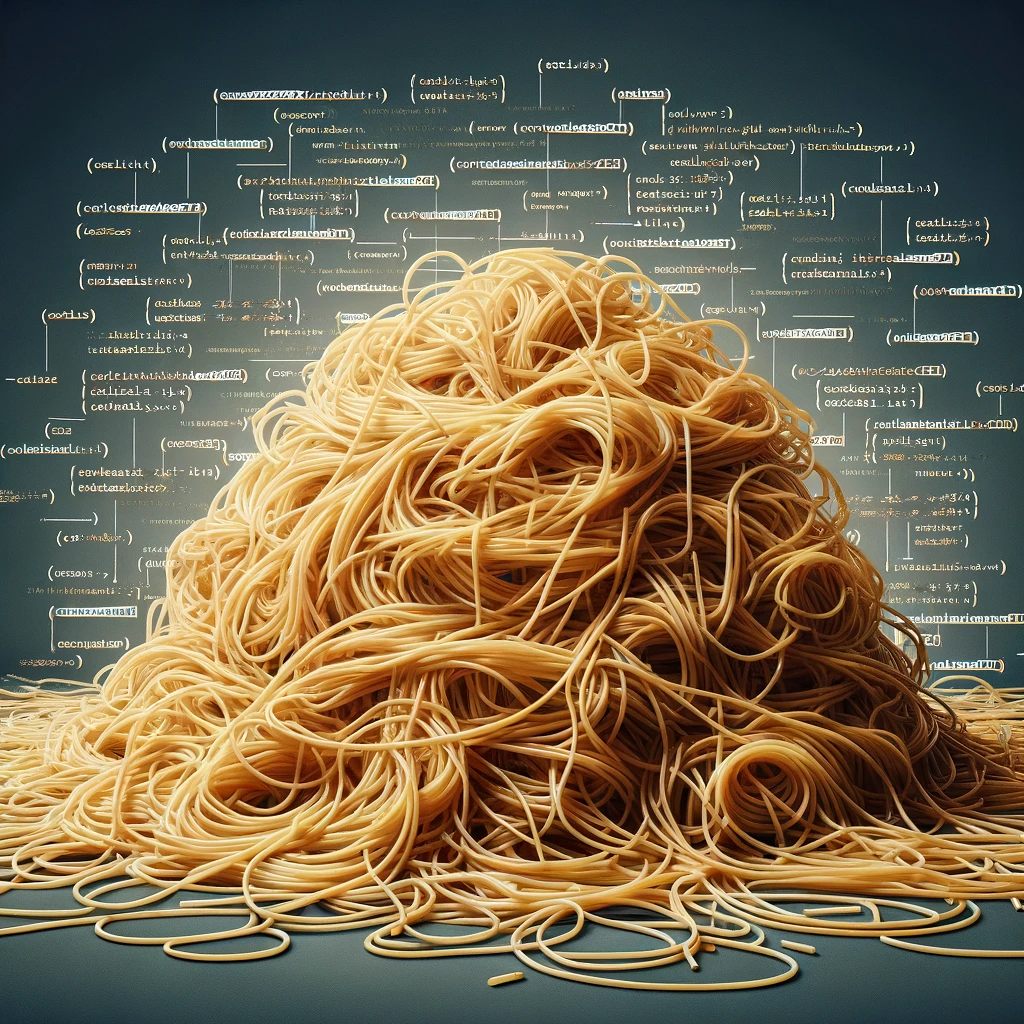
Refactoring isn’t a task you do in isolation; it’s a vital part of the software development process that intertwines with development cycles. I’ll present real-world case studies where refactoring propelled projects forward, yielding significant gains in efficiency and reducing technical debt.
“Spaghetti code” refers to a programming style characterized by a tangled, unstructured, and difficult-to-follow codebase. It typically arises from haphazard development practices, a lack of planning, or frequent modifications without proper refactoring. Here’s an example of spaghetti code in Python:
def calculate_total_price(items):
total_price = 0
# Loop through each item in the list
for item in items:
# Check if the item is in stock
if item['quantity'] > 0:
# Calculate the item price
if item['type'] == 'book':
if item['genre'] == 'fiction':
price = item['price'] * 0.9
elif item['genre'] == 'non-fiction':
price = item['price'] * 0.8
else:
price = item['price']
elif item['type'] == 'electronic':
if item['brand'] == 'Apple':
price = item['price'] * 1.2
elif item['brand'] == 'Samsung':
price = item['price'] * 1.1
else:
price = item['price']
else:
price = item['price']
# Update total price
total_price += price * item['quantity']
# Apply discount if total price is above $1000
if total_price > 1000:
total_price *= 0.9
return total_price
In this example:
- The code lacks clear structure and readability.
- The logic for calculating item prices is convoluted and nested, making it hard to understand and maintain.
- There are redundant conditions and calculations.
- The function’s purpose (calculating the total price of items) is buried within a maze of if statements and loops.
To improve this code, you could refactor it by breaking it into smaller, more manageable functions, simplifying conditional logic, and adding comments to explain the purpose of each section.
To refactor the spaghetti code into a more organized and readable structure, we can break down the logic into smaller, focused functions, simplify conditional checks, and improve code clarity. Here’s a refactored version:
def calculate_item_price(item):
price = item['price']
if item['type'] == 'book':
price = apply_book_discount(price, item['genre'])
elif item['type'] == 'electronic':
price = apply_electronic_markup(price, item['brand'])
return price
def apply_book_discount(price, genre):
if genre == 'fiction':
return price * 0.9
elif genre == 'non-fiction':
return price * 0.8
return price
def apply_electronic_markup(price, brand):
if brand == 'Apple':
return price * 1.2
elif brand == 'Samsung':
return price * 1.1
return price
def calculate_total_price(items):
total_price = 0
for item in items:
if item['quantity'] > 0:
total_price += calculate_item_price(item) * item['quantity']
return total_price
def apply_discount(total_price):
if total_price > 1000:
return total_price * 0.9
return total_price
items = [
{'type': 'book', 'genre': 'fiction', 'price': 10, 'quantity': 2},
{'type': 'book', 'genre': 'non-fiction', 'price': 15, 'quantity': 1},
{'type': 'electronic', 'brand': 'Apple', 'price': 100, 'quantity': 1},
{'type': 'electronic', 'brand': 'Samsung', 'price': 200, 'quantity': 1}
]
total_price = calculate_total_price(items)
final_price = apply_discount(total_price)
print("Total Price after discount:", final_price)
In this refactored version:
- Each function has a clear and single responsibility.
- Complex conditional logic is moved into separate functions for better readability.
- The main calculation logic is contained within the
calculate_total_price
function, which iterates over the items and calculates the total price. - The
apply_discount
function applies a discount if the total price exceeds a certain threshold. - By breaking down the code into smaller functions with clear names and responsibilities, we make it easier to understand, maintain, and extend.
The Golden Rules of Refactoring
Refactoring isn’t just about changing code. Adopting a methodology is essential to keeping the process manageable and the code integrity intact.

The principle of starting small is paramount. Tackle one problem at a time to avoid overwhelming the system or the developers. This approach minimizes risk and makes the changes easier to test and debug. Consider it the divide-and-conquer strategy of coding.
There’s a simple guideline that resonates with every seasoned developer: the Boy Scout Rule. It encourages you to leave the code better than you found it, making small enhancements with each opportunity. Consistent application of this rule can result in significant collective improvement over time.
Refactoring should always aim for clarity and simplicity. A common misconception is that complex code is somehow better or more sophisticated. In reality, the beauty of code lies in its readability and the ease with which others can understand and maintain it.
Finally, rigorous testing safeguards the integrity of code during the refactoring process. Implementing a robust testing framework ensures that any changes made don’t introduce new bugs and that the software continues to function as expected.
With these foundational rules in place, let’s consider how to approach the inevitable challenges of refactoring. Refactoring can seem daunting, especially when dealing with a large and complex codebase or balancing the need for new features.
Overcoming Common Refactoring Challenges
Embarking on a refactoring initiative isn’t without its hurdles. One daunting prospect is facing legacy code. Often large and without sufficient tests, this code can be a minefield for developers. The key here is not to overhaul everything overnight. Instead, I recommend identifying the most critical parts of the system that could benefit from refactoring and focusing on those areas first. It’s crucial to write tests for these segments before altering the code to ensure you’re not introducing new issues.
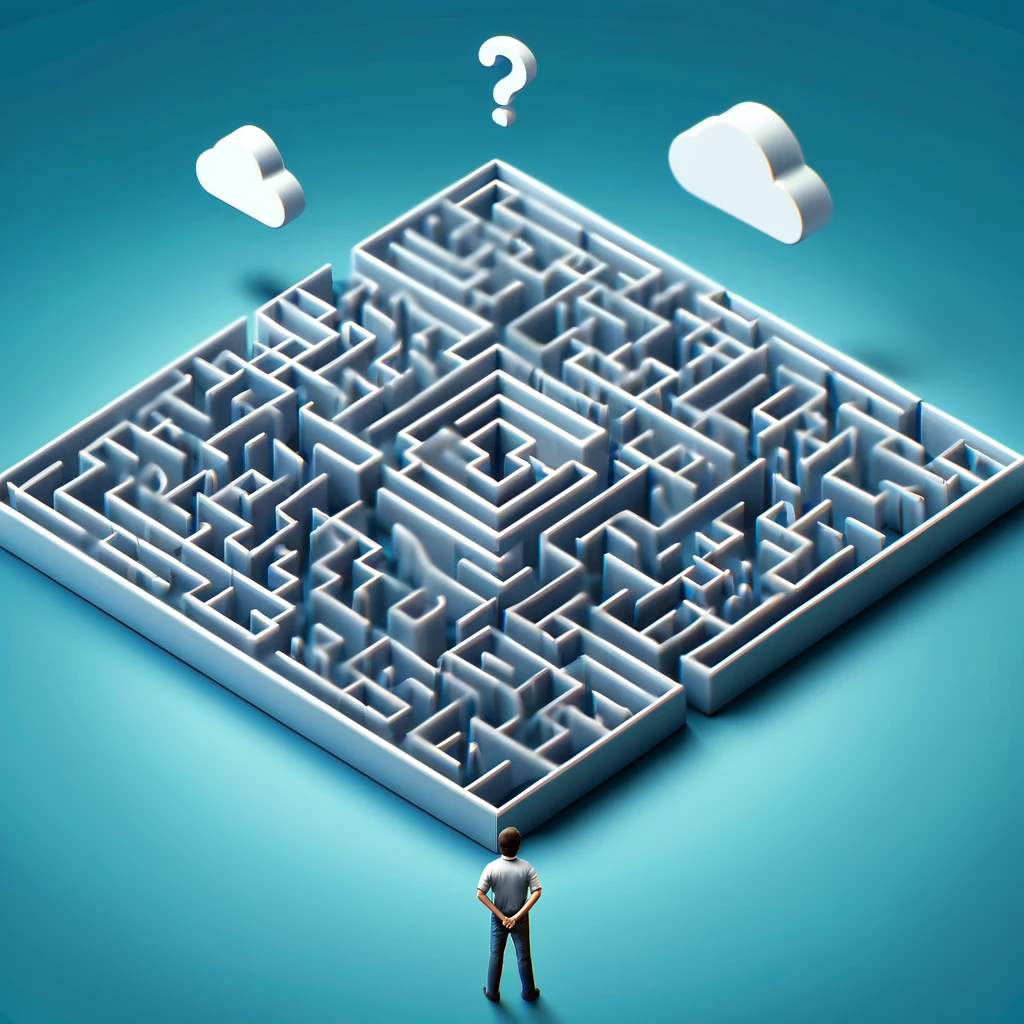
Another tug-of-war situation is balancing refactoring efforts with the pressure to roll out new features. It’s common for stakeholders to prioritize new developments over behind-the-scenes quality improvements. I’ve found that communicating the long-term benefits of refactoring, such as reduced maintenance costs and faster future development, can help align their expectations with the technical needs.
Clear communication with your team is just as essential. During refactoring, it’s easy for team members to step on each other’s toes. Transparency is vital. Use version control tools to manage changes efficiently and make sure everyone is aware of what’s being refactored. Regular meetings to discuss progress and challenges can maintain clarity and cohesion within the team.
Understanding that refactoring is not an end in itself but part of an ongoing process of improvement sets the stage for the next section. We’ll delve into real-world examples that underscore how best practices in refactoring are applied and bring tangible benefits to projects. More importantly, we’ll look at how you can measure the success of your refactoring efforts and ensure they contribute to a culture of continuous improvement.
Conclusion
Consistency is key, and I cannot stress enough the importance of making refactoring a habitual part of your workflow. Teams that thrive are the ones that merge refactoring with their daily development activities, ensuring CLEAN, MAINTAINABLE, and SCALABLE code is the standard, not the exception.
Read my other article about best practices.
Thank you for reading my post. Leave your comments below to participate in this engaging community. Be sure to sign up to receive updates of more incredible articles.
Share my article on social media and follow me on Twitter for extra content.
211 Followers
Jordan Devaney is a beacon in the tech world, guiding aspiring and seasoned developers alike through the complex landscape of web development and software engineering. Living in Whitmore Lake, Michigan, he combines his academic prowess, with degrees from Western Governor University and a Boot Camp certificate from Bloom Tech, with real-world experience to educate and inspire.
As the force behind DevTuneUp.com, Jordan offers a treasure trove of tutorials, how-tos, and insights into the ever-evolving tech industry. His role as a developer evangelist for nucamp further amplifies his commitment to tech education, making coding accessible to everyone keen to learn or switch careers.
With a sharp focus on demystifying technology and a goal to make DevTuneUp.com a cornerstone for tech enthusiasts, Jordan leverages his expertise in SEO optimization and social media marketing to reach and empower a global audience. Join him on this journey to navigate the digital world with confidence and skill.
To set up a 30 minute meeting via Google Meet and get resources about furthering coding eduction for beginners, click this calendy link.
Hello Jordan,
What stands out to me are the golden rules of refactoring that are outlined. Starting small, embracing the Boy Scout Rule, prioritising clarity and simplicity and rigorous testing are all foundational principles that ensure successful refactoring efforts.
The advice on overcoming common challenges, such as dealing with legacy code and balancing refactoring with new feature development, is incredibly practical and actionable. I look forward to learning more about this topic.
This was really interesting to read, the way you broke everything down makes things I once considered very confusing and complex to be a lot easier and simple to understand.
I like how you encourage taking things one step at a time, focusing on one problem before moving on to the next. I feel like you can’t go wrong with this approach in any aspect of your life, but it’s especially useful for more high-risk and complex situations like dealing with code.
Thank you for sharing this helpful article, I enjoyed reading it!
Hey Jordan.
As a developer myself, I find this post on code refactoring best practices to be incredibly enlightening and helpful. The importance of routine code refactoring to prevent code rot and improve maintainability is well-explained. I appreciate the emphasis on automating the refactoring process and the mention of tools like Eclipse and IntelliJ IDEA. The tips provided, such as writing tests and seeking code reviews, are practical and valuable for ensuring the success of refactoring efforts. Thank you for sharing this insightful resource!
Marios
Interesting read, Overall, your article has emphased the importance of code refactoring as an ongoing process that contributes to the long-term health and sustainability of software projects.
From my understanding, code refactoring is a critical process in software development aimed at improving the quality, maintainability, and efficiency of existing codebases. It involves restructuring code without changing its external behavior to make it more readable, understandable, and adaptable.
I look forward to reading the next post!
Thanks Jordan for making this information easy to understand for us not so tech savvy people. The visual representation of the spaghetti code and the refactored one really helped me understand the process. I enjoyed reading the post and learning so many new things.
The post was written so anyone can understand it and learn something new. Jordan made sure to clearly state the issue with the spaghetti code , the reasons why spaghetti code is a problem, and what needed to be done to fix the code.
The post also covered the golden rules of refactoring. Making sure to clarify that it’s not just about changing the code and every coder should follow the boy-scout rule leaving it better than you found it.
This post is full of information and is intended for anyone looking to learn more about code.